JavaScriptでつくるスクロールアニメーション
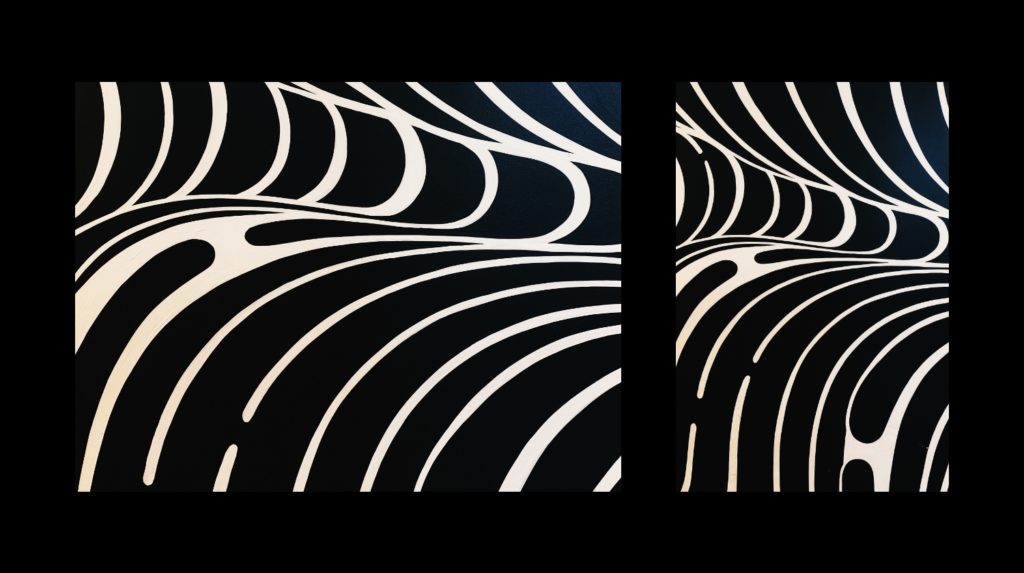
ブラウザをスクロールすると下からふわっと要素が出現するアニメーションを実装します。
JavaScriptで簡単に実装できますので、是非ご覧くださいませ。
サンプルをご覧ください
こちらのサンプルを下にスクロールしてみてください。
画像が下からふわっとアニメーション表示されたことが確認できたと思います。
今回は、このようなアニメーションを実装します。
HTMLの記述
<ul>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
<li class="js-scroll-animation"><img width="1600" height="900" src="https://unsplash.it/1600/900" alt=""></li>
</ul>
liタグにアニメーションを施しますのでjs-scroll-animation
クラスをつけます。
CSSの記述
ul{
display: flex;
flex-wrap: wrap;
}
li{
width: 50%;
padding: 20px;
box-sizing: border-box;
/* 透過・非表示 */
opacity: 0;
visibility: hidden;
/* 要素の位置指定・アニメーション */
translate: 0 20px;
transition: 1s;
}
img{
width: 100%;
height: auto;
}
/* クラス付与で表示する */
.is-active{
opacity: 1;
visibility: visible;
translate: 0 0;
}
liタグはデフォルトで「透過・非表示」と「要素の位置指定・アニメーション」のプロパティを指定します。
このスクロールアニメーションは要素にクラスを付与することで、下からふわっと出現する仕組みとなっています。「is-active」にクラスが付与されたときのプロパティを指定します。
JavaScriptの記述
//要素を取得
const animations = document.querySelectorAll('.js-scroll-animation');
function toggle() {
animations.forEach((animation) => {
//ウィンドウの高さを取得
const height = window.innerHeight;
//スクロール量を取得
const scroll = window.scrollY;
//要素の位置を取得
const target = scroll + animation.getBoundingClientRect().top;
//スクロール位置を判定して要素にクラスを付与
if (scroll > target - height / 1.5) {
animation.classList.add('is-active');
}
});
}
//ロード時に発火
window.addEventListener('load', toggle);
//スクロール時に発火
window.addEventListener('scroll', toggle);
document.querySelectorAll
で要素を取得- 要素は複数あるので
animations.forEach((animation)) => {}
で繰り返し処理 window.innerHeight
でウィンドウの高さを取得window.scrollY
でスクロール量を取得scroll + animation.getBoundingClientRect().top
で要素の位置を取得
あとは、if文で現在のスクロール量と要素の位置を比較して、要素にクラスを付与してアニメーションするようにしています。
まとめ
いかがだったでしょうか。
アニメーションの仕組みを理解すると簡単に実装することができましたね。
よかったら参考にしてみてください。